Renaming columns in Python
- Ekta Aggarwal
- Jan 28, 2021
- 1 min read
Updated: Jan 29, 2021
In this tutorial we will learn how to rename columns of a dataframe in Python.
Dataset:
In this tutorial we will make use of following CSV file:
Let us read our file using pandas' read_csv function. Do specify the file path where your file is located:
import pandas as pd
mydata = pd.read_csv("C:\\Users\\Employee_info.csv")
Let us create a copy of our dataset as mydata_copy.
mydata_copy= mydata.copy()
Renaming all the columns
We can fetch the column names as follows:
mydata_copy.columns
Output:
Index(['Emp Name', 'Sex', 'Dept', 'Salary', 'Rating'], dtype='object')
To rename all the columns we can assign a list of new column names as follows:
Note: The column names must be in exact order as the previous column names
mydata_copy.columns = ['Name','Gender','Dept','Salary','Rating']
mydata_copy.head(2)
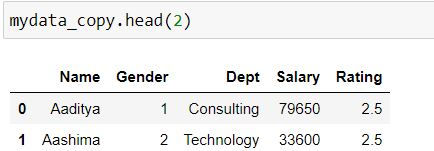
Renaming only some of the columns
To rename only some of the columns we use rename( ) function. In rename( ) we define columns = {our dictionary of key-values}
Keys are Old column names
Values are New column names
Let us rename columns Name and Gender to Emp Name and Sex respectively.
mydata_copy.rename(columns={"Name" : "Emp Name","Gender" : "Sex"})

By default inplace = False which means the changes won't be made in original dataset. Thus we need to save the new data.
To make changes in original data we set inplace = True.
mydata_copy.rename(columns={"Name" : "Emp Name","Gender" : "Sex"},inplace = True)
mydata_copy.columns
Output
Index(['Emp Name', 'Sex', 'Dept', 'Salary', 'Rating'], dtype='object')
Comments