Zip function and zipped objects
- Ekta Aggarwal
- Jan 26, 2021
- 2 min read
Zip function takes multiple iterator objects as input and returns a list of aggregated tuples as an output. It acts as a one to one mapping so that various objects can be linked together.
Syntax:
zip( iterator1 , iterator2, iterator3,... )
Topics covered:
Understanding with examples
Let us create 2 lists: product_category and their corresponding sales.
product_category = ['Biscuits','Lotions','Face creams','Cold Drinks']
sales = [5000,23400,30000,15000]
To link the two lists together we zip them and create a zipped_object:
zipped_object = zip(product_category,sales)
print(zipped_object)

To view the output the a zipped object we need to define it explicitly as a set or a tuple or a list:
list(zipped_object)
In the output first object from both the lists have been combined in first tuple ('Biscuits',5000), second object from both the lists are combined to form second tuple('Lotions',23400) and so on.
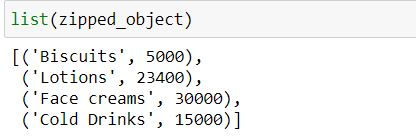
Zipping more than 2 objects:
Let us create 2 lists: product_category, their corresponding sales and returns.
product_category = ['Biscuits','Lotions','Face creams','Cold Drinks']
sales = [5000,23400,30000,15000]
returns = [1000,1300,2139,5839]
Let us zip them together
zipped_object = zip(product_category,sales,returns)
list(zipped_object)
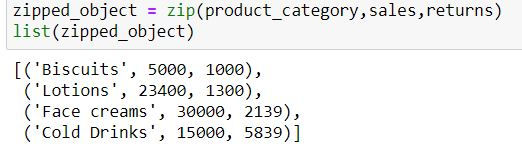
Iterator objects have unequal lengths
Let us consider a situation where we have only 3 product categories but sales and returns for 4 product categories.
product_category = ['Biscuits','Lotions','Face creams']
sales = [5000,23400,30000,15000]
returns = [1000,1300,2139,5839]
When we try to map such iterables of unequal length then the result is a zipped object has number of tuples = minimum length of iterable objects.
zipped_object = zip(product_category,sales,returns)
list(zipped_object)
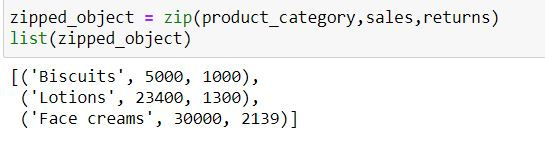
In our case since sales of 15000 and returns of 5839 can't be mapped to any product category thus hey have been dropped
Sorting the zipped objects:
Zipping the objects is extremely useful when we want to sort multiple objects on the basis of one single iterable.
Let us reconsider our 3 lists:
product_category = ['Biscuits','Lotions','Face creams','Cold Drinks']
sales = [5000,23400,30000,15000]
returns = [1000,1300,2139,5839]
Let us zip them together.
zipped_object = list(zip(product_category,sales,returns))
Using sorted function we can sort our zipped object.
Inside the sorted function we have defined a lambda expression x:x[0] which indicates that sorting should be done by first zipped iterable i.e. product_category
sorted_object = sorted(zipped_object,key = lambda x:x[0])
sorted_object
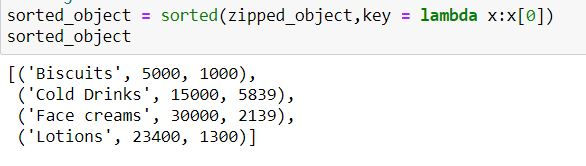
In the following lambda expression we have x : -x[1] indicating that sorting should be done in descending order by second zipped iterable i.e. Sales
sorted_object = sorted(zipped_object,key = lambda x:-x[1])
sorted_object
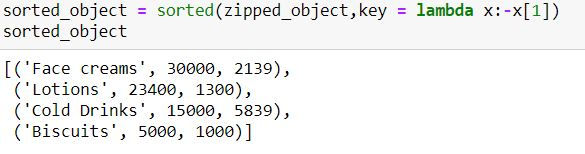
Simple x : -x[2] indicating that sorting should be done in descending order by third zipped iterable i.e. Returns
sorted_object = sorted(zipped_object,key = lambda x:-x[2])
sorted_object
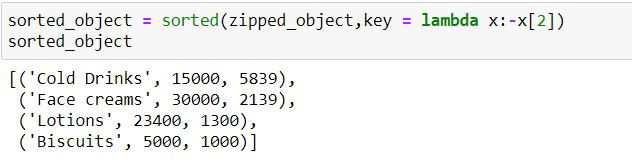
Looping over zipped objects:
We can iterate over multipe iterables in one single for loop using zipped objects (avoiding multiple for loops)
In the following code we have created two iterator objects pc and s which iterate over various values of zipped object one by one.
for pc,s in zip(product_category,sales):
print('Product category: ' + str(pc))
print('Sales: ' + str(s))
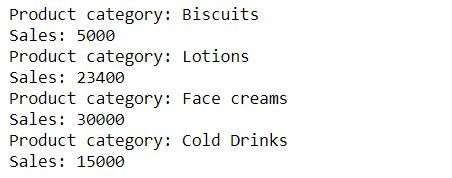
Unzipping objects
Zipped objects returns a list of tuples! To unpack them or unzip them we can use zip function, (There is no function called unzip :P)
Unzipping can be done by:
zip(* zipped object)
zipped_object
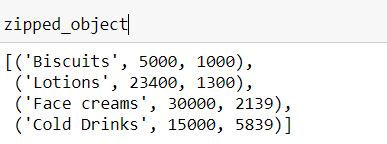
In the following code we are unzipping our zipped object using zip( *) and storing each element of the tuple in 3 different object.
Eg. from first tuple we have saved first element in unzipped_category, second element in unzipped_sales, and third element in unzipped_returns.
Similarly from second tuple each of the 3 element is saved in unzipped_category, unzipped_sales,unzipped_returns correspondingly.
unzipped_category, unzipped_sales,unzipped_returns = zip(*zipped_object)
print(unzipped_category)
print(unzipped_sales)
print(unzipped_returns)
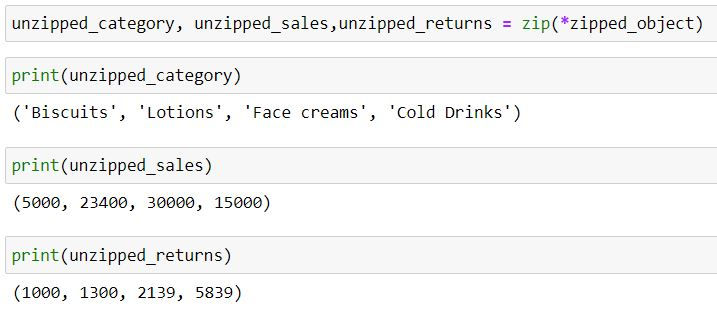
Comments